How to Scrape Amazon Prices Using Excel
If you’re here, you already know Amazon constantly tweaks product prices. The eCommerce giant makes around 2.5 million price changes daily, resulting in the average item seeing new pricing roughly every ten minutes. For sellers, marketers, and savvy shoppers, that creates both a challenge and an opportunity.
This comprehensive guide walks you through proven methods – from Excel's built-in tools to powerful scraping APIs that can simplify your Amazon price monitoring workflow.
Why track Amazon prices?
Price monitoring helps you stay informed and competitive. Here's why it matters:
- For Amazon sellers – Benchmark your prices against competitors to remain competitive
- For eCommerce businesses – Compare your pricing with Amazon's offerings to position your products effectively
- For marketing agencies – Analyze market trends and pricing patterns for client strategy development
- For individual shoppers – Receive alerts when prices drop on products you intend to purchase
To show the value of price tracking, here's a real-life example: A developer set up a simple Excel price tracker for a $1,500 gaming laptop. After monitoring for three weeks, they caught a 6-hour flash sale and saved $220 – a 15% discount that would have been impossible to find without automated price monitoring.
Method #1: using Excel Power Query
If you're new to web scraping (or just want the most straightforward solution) but are comfortable with Excel, Power Query is an excellent starting point. This tool lets you pull data from websites without any coding.
Step-by-step setup
- Open Excel and navigate to the Data tab.
- Click on Get Data > From Other Sources > From Web.
- Enter the URL of the Amazon product page you want to track.
- When the Navigator pane appears, look through the available HTML tables to find the one containing price information. Examine each to identify the one that includes details like discount, MRP, and current price.
- Click Transform Data to clean up the results by removing unnecessary rows and columns.
- Click Close & Load to import the data into your Excel sheet.
Here's what the final output looks like:
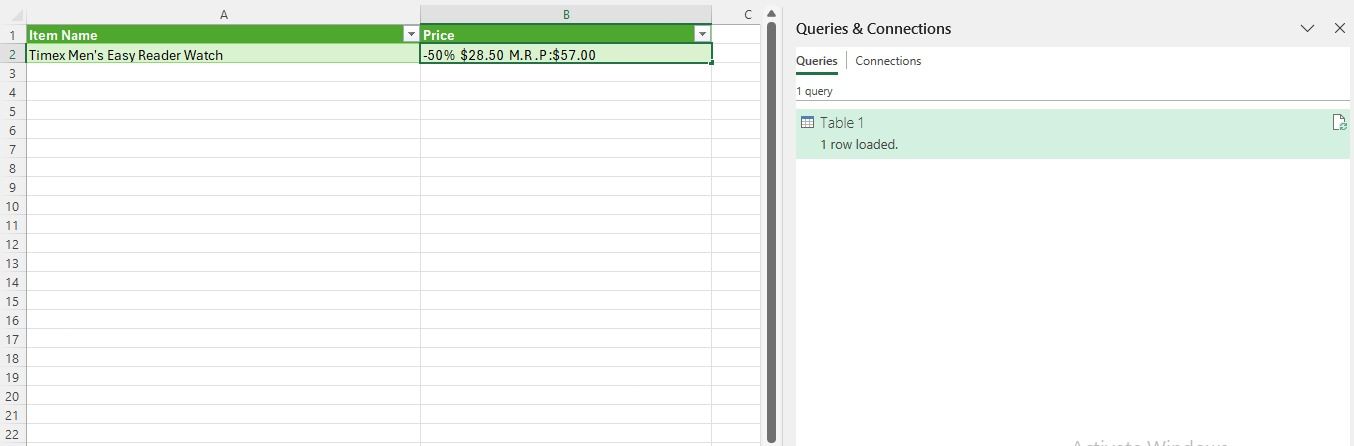
To update the prices later, simply right-click your query in the Queries & Connections pane and select Refresh.
Set up automatic refresh
To keep your price data fresh with minimal effort:
- Go to the Data tab and open the Queries & Connections pane (if it's not already visible).
- Right-click your query and select Properties.
- Check Refresh every and set your desired interval (minimum 1 minute).
- Optionally, check Refresh data when opening the file.
- Click OK to save your settings.
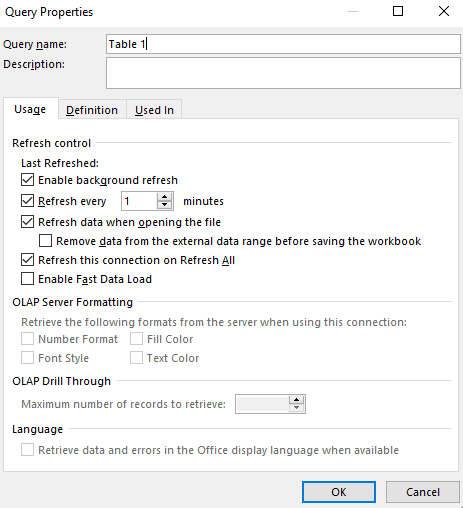
Method #2: using VBA (Visual Basic for Applications)
Visual Basic for Applications (VBA) gives you more flexibility and control when scraping Amazon prices. Here's how to implement this approach:
Set up your VBA environment
- Enable the Developer Tab in Excel: Go to File > Options > Customize Ribbon and check the Developer option.
- Open the VBA Editor by pressing Alt + F11.
- Insert a new module via Insert > Module.
- Paste the following code (replacing the URL with your product page):
Sub ScrapeAmazon()Dim HTML As Object, URL As StringDim ItemName As String, Price As StringURL = "YOUR_AMAZON_PRODUCT_URL"' Create HTTP requestWith CreateObject("MSXML2.XMLHTTP").Open "GET", URL, False.sendSet HTML = CreateObject("HTMLFile")HTML.body.innerHTML = .responseTextEnd WithOn Error Resume Next ' Handle missing elements gracefully' Extract item titleItemName = HTML.getElementById("productTitle").innerTextItemName = Trim(ItemName)' Extract pricePrice = HTML.getElementsByClassName("a-price-whole")(0).innerTextPrice = Trim(Price)' Output to ExcelRange("A1").Value = "Item Name"Range("B1").Value = "Price"Range("A2").Value = ItemNameRange("B2").Value = PriceOn Error GoTo 0End Sub
5. Run the script by pressing F5 to see the results.
Note: Amazon frequently updates its HTML structure, so you may need to adjust your selectors periodically. Use Chrome’s Developer Tools (right-click on the price and select Inspect) to find the correct selectors.
Learn more about how to choose the right selector for web scraping: XPath vs CSS.
Implement automatic refreshes with VBA
For true automation, create a VBA script that refreshes at regular intervals:
- Open the VBA Editor (Alt + F11).
- Insert a new Module and paste this code:
Dim NextScrapeTime As DoubleSub ScrapeAmazon()Dim HTML As Object, URL As StringDim ItemName As String, Price As StringURL = "YOUR_AMAZON_PRODUCT_URL"' Create HTTP requestWith CreateObject("MSXML2.XMLHTTP").Open "GET", URL, False.sendSet HTML = CreateObject("HTMLFile")HTML.body.innerHTML = .responseTextEnd WithOn Error Resume Next' Extract item titleItemName = HTML.getElementById("productTitle").innerTextItemName = Trim(ItemName)' Extract pricePrice = HTML.getElementsByClassName("a-price-whole")(0).innerTextPrice = Trim(Price)' Output to ExcelRange("A1").Value = "Item Name"Range("B1").Value = "Price"Range("A2").Value = ItemNameRange("B2").Value = PriceOn Error GoTo 0' Schedule next run in 2 minutesNextScrapeTime = Now + TimeValue("00:02:00")Application.OnTime NextScrapeTime, "ScrapeAmazon"End SubSub StopAutoScrape()On Error Resume NextApplication.OnTime NextScrapeTime, "ScrapeAmazon", , FalseEnd Sub
3. Double-click ThisWorkbook in the Project Explorer.
4. Paste this code to automate the process when opening and closing the workbook:
Private Sub Workbook_Open()ScrapeAmazonEnd SubPrivate Sub Workbook_BeforeClose(Cancel As Boolean)StopAutoScrapeEnd Sub
5. Save your file as an Excel Macro-Enabled Workbook (.xlsm)
Now, whenever you open the file, it will automatically start scraping every 2 minutes. To stop the cycle, either close the file or run the StopAutoScrape macro manually.
Limitations of Excel-based scraping methods
Keep in mind these significant limitations with Excel-based approaches:
- JavaScript rendering – Amazon loads many elements (including prices) with JavaScript after the initial page load. Since Excel can't execute JavaScript, it sometimes captures the price correctly, while other times, it fails.
- Anti-bot protection – Amazon employs sophisticated anti-scraping measures that can detect and block automated requests. This significantly reduces success rates for intensive scraping operations.
- Dynamic HTML structure – Amazon frequently updates its page structure, which breaks scrapers that rely on specific element selectors. This requires regular maintenance to keep your scrapers functioning.
- IP blocking – Repeated requests from the same IP address can trigger CAPTCHAs or blocks, especially when scraping at scale.
For these reasons, if you're serious about monitoring Amazon prices reliably, particularly at scale, you'll need a more robust solution.
Alternative solutions
If you're looking for different approaches to Amazon price tracking, consider these options:
Specialized price tracking tools
Several dedicated services exist specifically for Amazon price monitoring:
- Keepa – offers excellent visual data and detailed historical charts, though exporting data and analyzing it at scale can be challenging
- CamelCamelCamel – a free, functional tool with clear historical data and alert features, but its interface can be less intuitive, with reports that are sometimes hard to interpret and alerts that may lack accuracy
- Honey – best known for finding coupons, it provides basic price tracking; however, it lacks the depth and advanced features needed for detailed price analysis
While these tools are ideal for casual price tracking, they may not meet the needs of users looking for customization, scalability, or seamless data-sharing options for team-based or advanced analysis.
Using Python for custom solutions
For developers who prefer building custom solutions, Python offers robust libraries for web scraping:
- Beautiful Soup – for parsing HTML content
- Python Requests – for handling HTTP requests
- Selenium – for browser automation and JavaScript rendering
- Playwright – a newer alternative to Selenium for complex web scraping
For production-level monitoring, you'll want to implement rotating proxies and follow various web scraping best practices to prevent IP blocks and improve the overall success rate from Amazon.
Google Sheets
If you prefer using Google Sheets instead of Excel, you can also implement Amazon price tracking there. Learn more in this detailed guide on Amazon price scraping with Google Sheets.
Easiest solution: Smartproxy eCommerce Scraping API
Smartproxy's eCommerce Scraping API offers a surefire way to scrape Amazon undetected and without limitations. When reliability and scale become priorities, this purpose-built scraping API is the most effective option. Smartproxy's eCommerce Scraping API handles all the technical challenges of Amazon price scraping, including:
- JavaScript rendering – ensures all dynamically loaded price data is captured
- Proxy rotation – prevents IP blocks by distributing requests across different addresses
- CAPTCHA solving – automatically handles verification challenges
- Global coverage – tracks prices from any Amazon locale worldwide
- Structured data output – delivers clean, organized data in JSON or CSV format
Get started with Smartproxy's eCommerce Scraping API
- Create an account at Smartproxy.
- Navigate to the dashboard > Scraping APIs > Pricing.
- Select eCommerce in the target group and choose a subscription type (either Core or Advanced plans).
- Toggle Start with trial to access a 7-day free trial before full commitment.
- Return to the dashboard and select your plan (eCommerce Advanced or eCommerce Core) under the Scraping APIs section.
- Choose Amazon Pricing Scraper as your target.
- Enter the product ASIN you want to track.
- Check the Parse checkbox to receive structured data.
- Configure additional parameters if needed.
- Send the request.
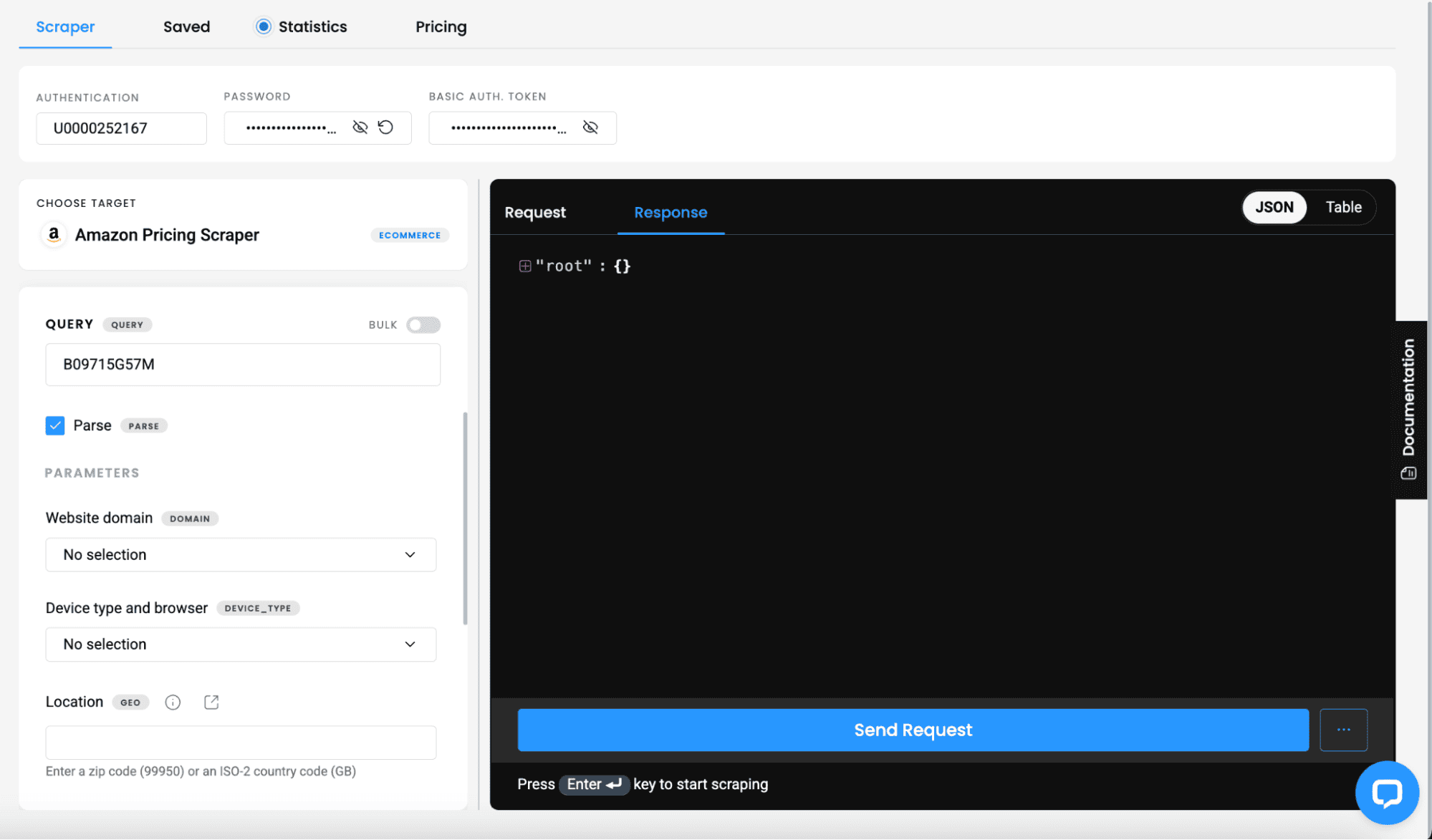
The API returns clean, structured data that you can export as CSV or JSON:
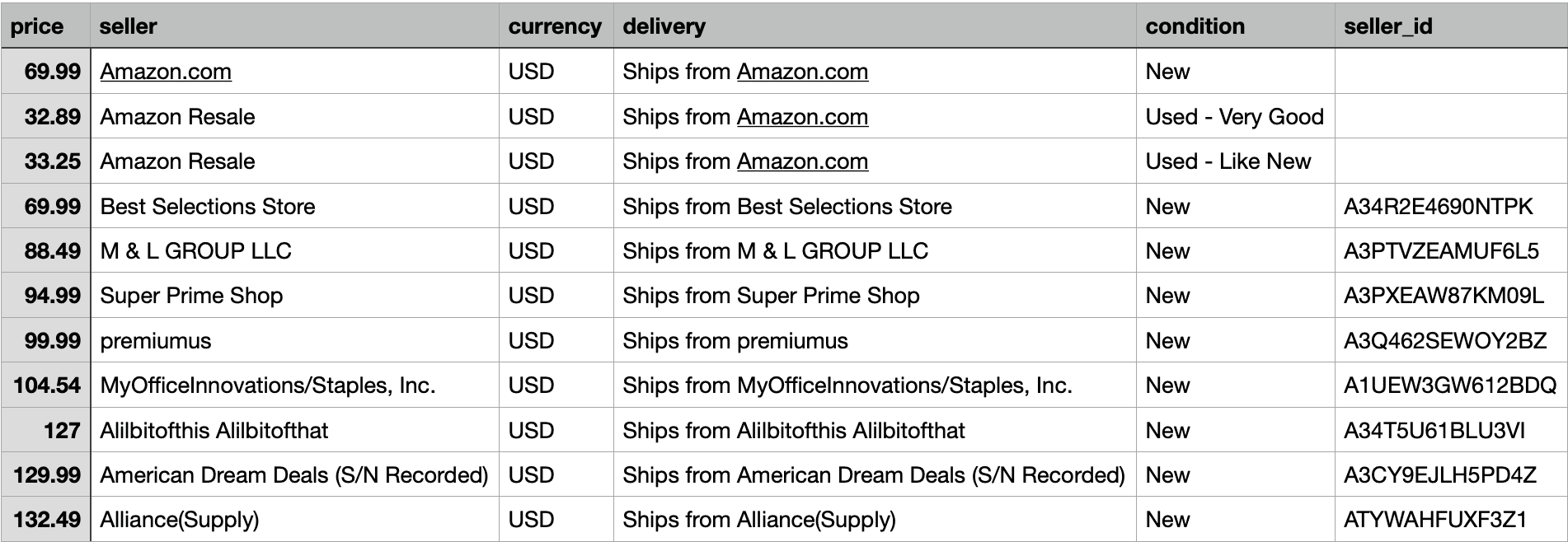
If you prefer coding, here's how to use the API with Python:
import requestsurl = "https://scraper-api.smartproxy.com/v2/scrape"payload = {"target": "amazon_pricing","query": "B09715G57M", # Replace with your product ASIN"page_from": "1","parse": True,}headers = {"accept": "application/json","content-type": "application/json","authorization": "Basic SMARTPROXY_AUTH_TOKEN", # Replace with your actual token}response = requests.post(url, json=payload, headers=headers)print(response.text)
Let's break down the parameters:
- target – inform the API that we want Amazon price data
- query – this is the ASIN (Amazon Standard Identification Number). Replace this with the ASIN of the product you want to scrape
- page_from – specifies the search result page to scrape
- parse – enables automatic parsing, meaning the API will return structured data instead of raw HTML
The API supports multiple parameters beyond those shown above. For a complete list, refer to the official parameter list.
Important: Remember to replace SMARTPROXY_AUTH_TOKEN with your actual token from the dashboard.
The API returns structured JSON data:
{"results": {"url": "https://www.amazon.com/gp/aod/ajax/ref=dp_aod_unknown_mbc?asin=B09715G57M&pageno=1","asin": "B09715G57M","page": 1,"title": "Keurig K-Express Single Serve K-Cup Pod Coffee Maker, 3 Brew Sizes, Strong Button Feature, 42oz Removable Reservoir, Black","pricing": [{"price": 69.99,"seller": "Amazon.com","currency": "USD","delivery": "Ships from Amazon.com","condition": "New",// additional data...},// more seller listings...]}}
If you prefer a different programming language, head to the dashboard and customize the parameters there. Smartproxy automatically generates the code in various languages, including Node.js, cURL, and Python.
Want to continue exploring the advanced settings of our Scraping APIs? Explore our friendly documentation.
Method comparison: which approach is right for you?
Among all the Amazon scraping methods discussed, each has distinct advantages and limitations. Here's a comparison to help you choose the right approach for your specific needs:
Excel Power Query
VBA Macros
Smartproxy API
Reliability
Unreliable
Unreliable
100%
Scale (# of products)
Very limited
Limited
Unlimited
Anti-blocking features
None
Limited
Comprehensive
JavaScript handling
Poor
Poor
Excellent
Data structure
Manual cleanup needed
Basic extraction
Fully structured
Maintenance required
High
High
None
Cross-platform
Windows only
Windows only
Any OS/platform
Setup difficulty
Easy
Moderate
Easy
Wrapping up
We've covered multiple ways to monitor Amazon prices – from simple Excel tools to robust scraping APIs. While the Excel methods work for basic tracking, they quickly hit limitations when scaling. Amazon's frequent security updates make consistent price tracking increasingly challenging.
For reliable, hassle-free price monitoring at scale, Smartproxy's eCommerce Scraping API does the heavy lifting – handling anti-bot measures and delivering clean pricing data without the maintenance headaches.
About the author
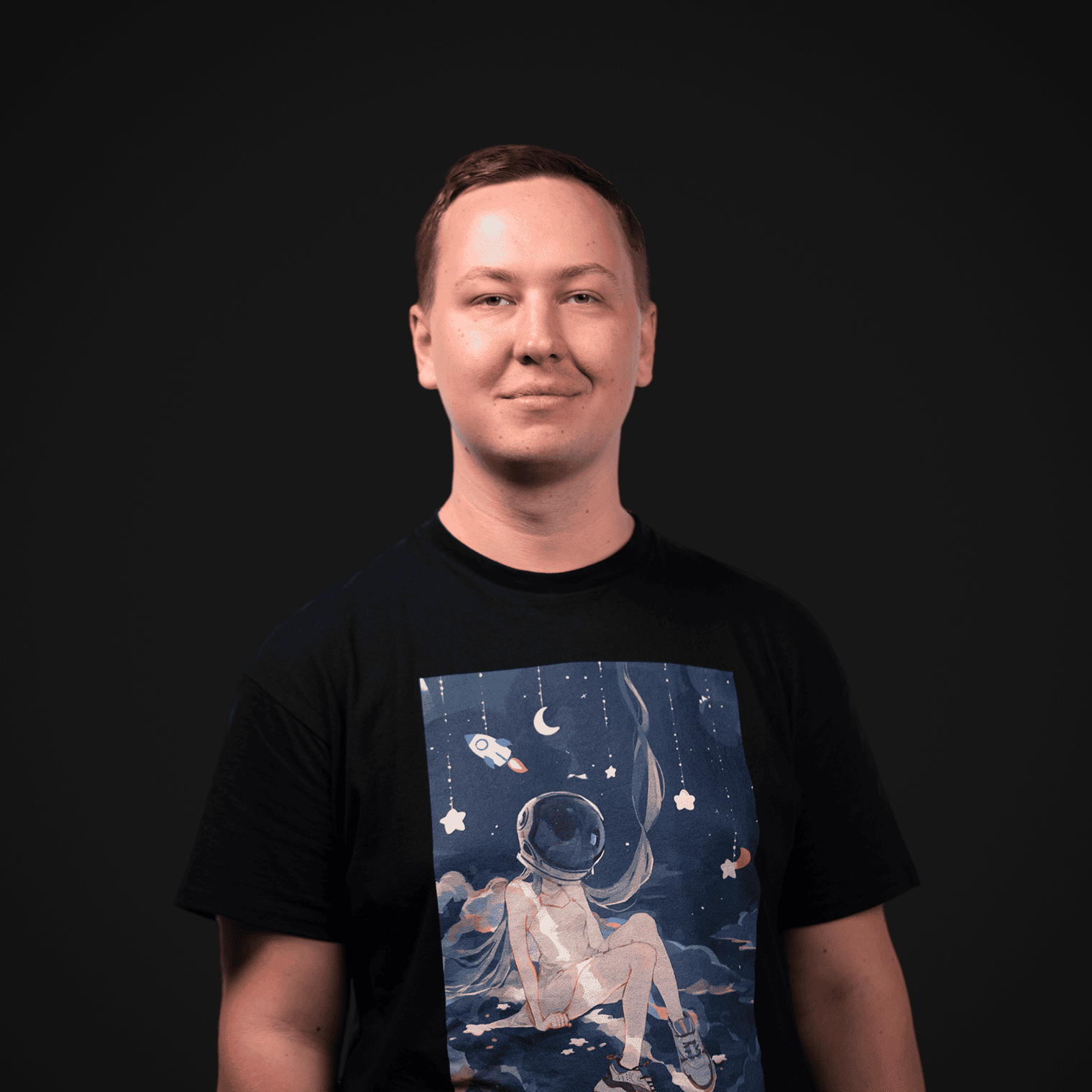
Zilvinas Tamulis
Technical Copywriter
A technical writer with over 4 years of experience, Žilvinas blends his studies in Multimedia & Computer Design with practical expertise in creating user manuals, guides, and technical documentation. His work includes developing web projects used by hundreds daily, drawing from hands-on experience with JavaScript, PHP, and Python.
Connect with Žilvinas via LinkedIn
All information on Smartproxy Blog is provided on an as is basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Smartproxy Blog or any third-party websites that may belinked therein.