Mastering Python Requests: A Comprehensive Guide
Python’s Requests library is one of the most powerful web tools. Find out what it is, how to use it, and how to make the most out of it with Smartproxy proxies.
The importance of Python Requests
Requests is a tool that enables Python developers to communicate with the web through HTTP requests. It provides a simple and elegant API that simplifies the process of making GET and POST requests and other methods of communication with a web server. It’s a popular Python library, with over 300 million monthly downloads and 50K+ stars on GitHub, making it one of the most reliable and trusted tools out there. Requests solidifies itself in the developer community as a popular library for several reasons:
- It’s simple and easy to use. If you were to write requests manually, you’d end up with large bits of code that are hard to read, maintain, and understand. Requests simplify the process, reducing the code you must write for complex tasks.
- The Python Requests module has many features that simplify the developer's life – a consistent interface, session and cookie persistence, built-in authentication support, and content parsing to data structures such as JSON. These are only a few of the things that Requests offers as a library, and there are no limits on what it can do – it’s extensible for more advanced use cases and scenarios, so you can be sure you’ll never run into a dead-end.
- Finally, Requests features proxy support, allowing you to integrate Smartproxy proxies easily. A proxy enhances security by making requests appear from different locations and IP addresses. This is incredibly useful for tasks such as web scraping or automation tools that might run into risks of being rate-limited or IP-banned by certain websites if too many requests are made from the same device.
Getting started
To get started with the Python Requests library, you only need to have the latest version of Python on your computer. Then, run the following command in your Terminal to install the Requests package:
pip install requests
If you get the "error: externally-managed-environment" after running the above line, check out our solution. Once installed, you can use it in your code simply by including this line at the beginning:
import requests
A GET request is a method used to retrieve data from a specified resource on a server, typically by appending parameters to the URL. Here’s a simple code example that makes a request to test if our library works:
import requestswebsite = "https://ip.smartproxy.com/json"response = requests.get(website)print(response.content)
To run it, navigate to the project folder in your Terminal and enter the following command:
python file_name.py
That is the beauty of Requests. Just like that, with barely a few lines of code, we made a request to a target website and printed its content.
Proxy integration
Before moving any further, we need to add a critical spice to our dish – some delicious Smartproxy proxies. They’re an essential part of the code, as websites will often employ serious anti-bot protection, and any automated requests will likely be met with restrictions. Let’s stay one step ahead of the game and set up some proxies in our code.
To begin, head over to the Smartproxy dashboard. From there, select a proxy type of your choice. You can choose between residential, ISP, and datacenter proxies. We’ll use residential proxies for this example:
- Find residential proxies by navigating to Residential under the Residential Proxies column on the left panel, and purchase a plan that best suits your needs.
- Open the Proxy setup tab.
- Head over to the Endpoint generator.
- Click on Code examples.
- Configure the proxy parameters according to your needs. Set the authentication methodauthentication method, location, session typesession type, and protocolprotocol.
- Further below in the code window, select Python on the left.
- Copy the code.
These steps will provide you with an example code snippet that conveniently also uses the Requests library:
# The code might appear slightly different from this one according to the parameters you've setimport requestsurl = 'https://ip.smartproxy.com/json'username = 'user'password = 'pass'proxy = f"http://{username}:{password}@gate.smartproxy.com:10000"result = requests.get(url, proxies = {'http': proxy,'https': proxy})print(result.text)
This code makes a simple request to https://ip.smartproxy.com/json, which returns information about your location and IP address in JSON format. You can be sure that the proxies work because they’ll show a different location and IP address than your current one.
POST requests
Similar to GET requests, you can also send POST requests. A POST request is a method to submit data to be processed to a specified resource on a server. In contrast to GET requests that retrieve data, POST requests typically involve sending data in the request body, often used for tasks like form submissions or uploading files.
Here’s an example code to send a POST request to a target website with data:
import requestsurl = 'https://httpbin.org/post'username = 'user'password = 'pass'proxy = f"http://{username}:{password}@gate.smartproxy.com:10000"# Data for the POST requestdata = {'name': 'value1', 'key2': 'value2'}result = requests.post(url, data=data, proxies={'http': proxy, 'https': proxy})# Print the response contentprint(result.text)
This script sends a POST request to a sample website httpbin, which returns a response upon success:
{"args": {},"data": "","files": {},"form": {"key1": "value1","key2": "value2"},"headers": {"Accept": "*/*","Accept-Encoding": "gzip, deflate","Content-Length": "23","Content-Type": "application/x-www-form-urlencoded","Host": "httpbin.org","User-Agent": "python-requests/2.31.0"},"json": null,"origin": "xxx.xxx.xx.xxx","url": "https://httpbin.org/post"}
The data we sent appears under “form”, indicating that the data was passed to the server. You can also check the IP address next to “origin” to ensure that this request was also made from a different IP address from your own.
Basic authentication
Sometimes, to communicate with a server, you’ll need to provide credentials to connect to it. Here’s a basic authentication example that will make a request while also sending the username and password to the server:
import requestsurl = 'https://ip.smartproxy.com/json'proxy_username = 'proxy_user'proxy_password = 'proxy_pass'auth_username = 'your_username'auth_password = 'your_password'proxy = f"http://{proxy_username}:{proxy_password}@gate.smartproxy.com:10000"result = requests.get(url, proxies = {'http': proxy,'https': proxy})general_auth_credentials = requests.auth.HTTPBasicAuth(auth_username, auth_password)result = requests.get(url, proxies={'http': proxy,'https': proxy,'auth': general_auth_credentials})print(result.text)
Status codes
When you make any kind of an HTTP request, you’ll run into a status code that tells you the status of your request and whether it was successful or not. To learn more about what the status code you’ve received means, check out our comprehensive documentation that lists the explanations of the most common responses.
Use cases
Python’s Requests can be applied for many uses, either independently or with other tools or libraries. Here are some cool examples:
- API requests – many web applications offer intuitive API interfaces to interact with them. If you’re going to build an application of your own that relies on the information and content another one provides, accessing their API and interacting with them is extremely important. For example, you’re building a Python app that tells users about the latest music trends, the most popular songs this week, etc. You’ll need to access the APIs of many streaming platforms and retrieve data about how often songs were played, artist and track names, album covers, etc.
- Handling responses – when you make an HTTP request to a web server, it will always provide one of many responses. This will be an HTTP response status code, a way for the server to tell the status of your HTTP request, whether it was successful, failed, or something happened in between. If your code interacts with a web service often, it will likely run into one of these HTTP errors eventually, especially if it makes multiple requests from the same IP address and gets rate-limited or blocked. It’s crucial to identify when these errors happen and modify your code to react accordingly to the response type it receives.
- Web scraping – one of the most common reasons to write scripts that search the web for you is for data collection, otherwise known as scraping. It’s a process of making an HTTP request to a web page, extracting all the data from it, and then parsing it or saving it for analysis later. Information like this is later used for various research purposes, market intelligence, competitor analysis, price comparison, and many other reasons.
Proxy performance and security benefits
Smartproxy proxies are essential to using the Requests library as they enhance its functionality. Proxies enhance speed and reliability and prevent your code from running into common issues when running scripts on the web.
The most important thing to remember is that a regular user using a browser to check websites will usually not run into any issues doing so. Some of their actions may raise suspicions, but they will most likely get resolved quickly, and no danger flags will be raised. Meanwhile, an automated script is a wild, untamed beast that doesn’t follow any standard procedures of a regular user, and websites will quickly recognize it, try to stop it, and put it back in a cage.
While it’s completely understandable that websites implement measures against potentially harmful bots, not all scripts are malicious; yet they face the same limitations and consequences.
Proxy services come in handy when trying to circumvent these limitations, as they allow you to make requests from different IP addresses while maintaining your anonymity. This way, websites don’t know if the requests come from the same or multiple sources, making limiting or blocking harder.
Smartproxy offers a wide range of proxy solutions to use together with Requests. You can choose from many proxy types and pick from a massive pool of 65M+ IPs across 195+ locations worldwide with unlimited connections and threads and fast response times. These features make your automated script actions on the web more secure and more challenging to detect with no cost to performance or having to make massive changes to your code or infrastructure.
Final thoughts
Python’s Requests remains one of the most popular choices for many web-based applications, and it doesn’t look like that will change any time soon. It’s a crucial tool at your disposal and will be a base for many of your web scraping scripts. The best part about it is that it’s super simple to get started, and paired together with Smartproxy proxies, it becomes an unstoppable force that will easily overcome any difficulties.
About the author
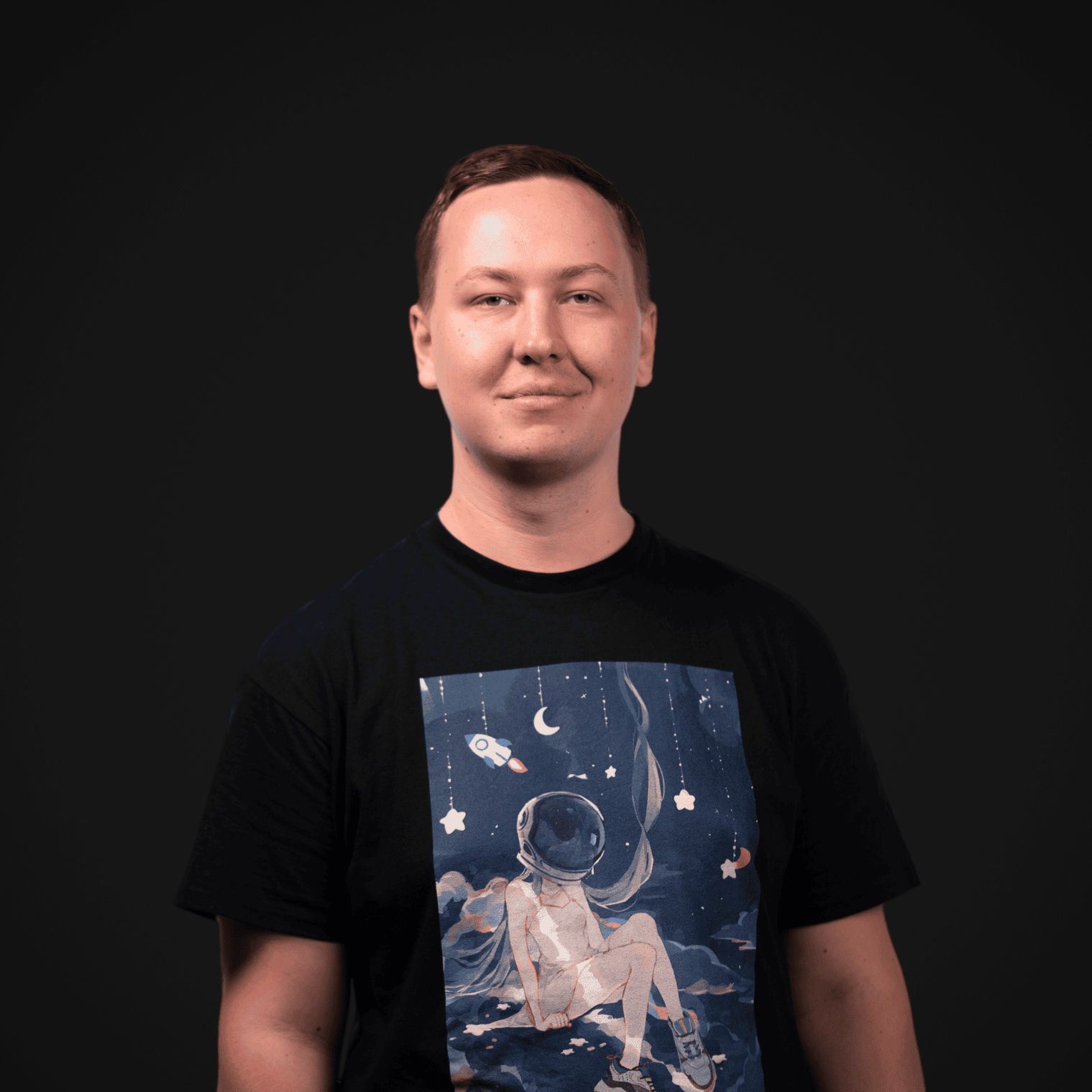
Zilvinas Tamulis
Technical Copywriter
A technical writer with over 4 years of experience, Žilvinas blends his studies in Multimedia & Computer Design with practical expertise in creating user manuals, guides, and technical documentation. His work includes developing web projects used by hundreds daily, drawing from hands-on experience with JavaScript, PHP, and Python.
Connect with Žilvinas via LinkedIn
All information on Smartproxy Blog is provided on an as is basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Smartproxy Blog or any third-party websites that may belinked therein.