What to do when getting parsing errors in Python?
This one’s gonna be serious. But not scary. We know how frightening the word “programming” could be for a newbie or a person with a little technical background. But hey, don’t worry, we’ll make your trip in Python smooth and pleasant. Deal? Then, let’s go!
Python is widely known for its simple syntax. On the other hand, when learning Python for the first time or coming to Python after having worked with other programming languages, you may face some difficulties. If you’ve ever got a syntax error when running your Python code, then you’re in the right place.
In this guide, we’ll analyze common cases of parsing errors in Python. The cherry on the cake is that by the end of this article, you’ll have learnt how to resolve such issues.
What are parsing errors?
In linguistics, syntax defines the grammatical rules of spoken languages. Similarly, syntax in computing deals with a set of rules for programming languages. These rules indicate what is valid in a particular programming language and what it needs to be functional and readable.
Syntax is checked by an interpreter. It’s a computer program that parses initial code to convert it into machine code. Then, the interpreter executes instructions written in a programming or scripting language.
So parsing errors are mistakes in code that don’t comply with Python rules. When you run your code in Python, it’s the interpreter that checks your code and looks for any parsing errors that you might have left in your code. It acts like a teacher at school, doesn’t it?
What is SyntaxError?
Interpreters detect various types of errors, two of which are most prominent: syntax (or parsing) errors, and exceptions. There’s a subtle difference between the two: you can’t handle syntax errors at the run time, while Python exceptions can be dealt with.
You might refer to syntax errors and resulting exceptions as invalid syntax. So how does a parsing error appear? Pretty straightforward answer here: when a programmer breaks Python syntax, the interpreter identifies a mistake and marks it as invalid syntax.
In such cases, Python raises a SyntaxError exception and provides a traceback, which includes clues on how to debug the error. Look at this code block and its SyntaxError traceback:
12 x = 1034 y = 556 sum = x y
λ python invalid_syntax__no_operator.pyFile "invalid_syntax_no_operator.py", line 6sum = x y^SyntaxError: invalid syntax
There are a few elements of a SyntaxError traceback that show where the interpreter has found the invalid syntax in your code:
- The file name – shows in which file the invalid syntax arose.
- The line number & the reproduction of that line – indicates the number of the line where the issue occurred and reproduces the whole line.
- A caret (^) – this arrow is always on the line below the reproduced code and specifies the concrete element in the code that has a problem.
- The error message – comes last, after the words “SyntaxError,” and provides information on the type of problem.
In the example above, the file name is “invalid_syntax_no_operator.py” and the line number is 6. The caret points to the “y” in “sum = x y” and the error message is “invalid syntax.” Although a SyntaxError traceback might not indicate the real problem, it points to the first thing of syntax that doesn’t make sense for the interpreter. The correct version would be “sum = x + y.”
To sum up, tracebacks or SyntaxErrors appear when the Python interpreter doesn’t get what the programmer has asked it to do. When a SyntaxError like this one occurs, the program ends immediately because it can’t understand what the next execution should be. Then, you must change the syntax of your code and rerun the program.
Datacenter proxies now up to 74% off
Access 500K+ shared and dedicated datacenter proxies in global locations.
Common Python syntax errors
Did you ever encounter a SyntaxError while coding? Wasn’t that quite frustrating? Yet, no stress! We’re gonna show you the most common reasons that cause syntax errors and how to fix them.
Missing the semicolon
Most probably, developers make this type of error most often. This might be the case as a semicolon is a must if you want to terminate the line of code in programming languages like C, C++, and Java.
However, this isn’t true in Python. A semicolon in Python is a must if you wish to write multiple statements in a single line. So in Python, a semicolon denotes separation, not termination.
If you miss this punctuation mark, the interpreter won’t understand different statements and won’t be able to execute your code. This, in turn, leads to a syntax error.
In the example below, the caret shows that line 5 in your code lacks a semicolon after the closing bracket.
1 customers = [2 {"name": "Mary", "lname": "Stewart"},3 {"name": "John", "lname": "Doe"},4 {"name": "Joseph", "lname": "Anderson"},5 ]67 for c in customers: print ("Name: ") print (c['name'])
λ python missing_semicolon.pyFile "missing_semicolon.py", line 7for c in customers: print ("Name: ") print (c['name'])^SyntaxError: invalid syntax
The correct code in line 7 should look like this:
for c in customers: print ("Name: ") ; print (c['name'])
Misusing the assignment operator
As the name suggests, the assignment operator (=) assigns the value of the right side of an expression to the left side. Not using it results in invalid syntax. Let’s take a test. In the code block below, look at lines 2, 4, and 6 – is everything okay with them?
2 a = 7234 b = 5456 x a + b
If you said no, congrats! The code block does include a mistake. Such kind of coding will return the following SyntaxError traceback:
λ python missing_assignment_operator.pyFile "missing_assignment_operator.py", line 6x a + b^SyntaxError: invalid syntax
Here we see that there’s something wrong with line 6. If you come back to it in the upper code block, you’ll see that the “x a + b” part does lack something. In this case, we’re missing the assignment operator, showing that the value of “x” equals the sum of “a” and “b”:
x = a + b
The SyntaxError message may not seem extremely helpful in this case. It doesn’t clearly say that an assignment operator is missing. Yet, every programmer, even a beginner, will recognize that they’ve made this type of mistake here.
Apart from this, the assignment operator has more functions than just assigning values. For example, if you write two equal signs one after another (==), this will turn an assignment into a comparison.
Using parentheses, brackets, and quotes
A parenthesis is a punctuation mark that we use to enclose information. Forgetting to close a string in a parenthesis occurs quite often, too. And this includes both double-quoted (“don’t”) and single-quoted (‘do’) strings.
Okay, one more test for you. Look at the code with a parenthesis below and notice if you see something bad:
10 list = ["item1", "item2", "item3", "item4", "item5]
Did you recognize that the last quotation mark is missing? As a result, the EOF error will be thrown:
λ python eof2.pyFile "eof2.py", line 10list = ["item1", "item2", "item3", "item4", "item5]^SyntaxError: EOL while scanning string literal
This time, the SyntaxError message is “EOL while scanning string literal.” EOL stands for “end of line” and means that the Python interpreter ran to the end of a line before an open string was closed. To fix this issue, close the string with the quotation mark you used to start it. In this case, you would need to use a double quote ("):
list = ["item1", "item2", "item3", "item4", "item5"]
You can spot this quite easily in short codes but you might face some issues if your code is a long line consisting of many elements. Trust us, this is particularly tough in longer multi-level parentheses that have many brackets. Consider this:
14 product_name = "Sony Playstation 5"15 product_price = 4001617 print ("Product: {} costs ${}"(product_name, product_price)18
Do you see what’s wrong here? Need some help? Have a look at the traceback that was produced after writing that code:
λ python eof3.pyFile "eof3.py", line 18SyntaxError: unexpected EOF while parsing
This isn’t really helpful, is it? There is no caret, nor is there any specific issue indicated, just “unexpected EOF while parsing.” The point is that the interpreter knows that there is a missing bracket but can’t detect the exact place where it should be used.
The key here is EOF, which stands for “end of file.” It means that the Python interpreter read the whole code until the end (the end of this code is line 18, indicated by the EOF message), spotted that somewhere there’s a problem with the code, and returned a syntax error related to an unexpected EOF while parsing.
A programmer would need to review the code manually from the very beginning, insert the missing bracket, and run the program again. The correct version of the aforementioned code is this:
print ("Product: {} costs ${}"(product_name, product_price))
Missing the comma
Oh yes, those small tiny pieces at the bottom of a line… The thing is that Python doesn’t have a comma operator, while the C and C++ programming languages do. No wonder missing a comma is one of the most common Python syntax errors.
The only place in Python code where commas might look like operators is in tuple literals. A tuple is a string of elements that are surrounded by brackets and separated by commas. For instance, (2, 7, 4, 1, 7) is a 5-tuple.
Let’s have a look at this code with tuple literals. Try to find the place where a comma should be placed:
4 customers = [5 {"name": "Mary", "lname": "Stewart"},6 {"name": "John", "lname": "Doe"}7 {"name": "Joseph", "lname": "Anderson"},8 ]9 10 for customer in customers:11 print (f"Name: {customer['name']}")12 print (f"Last Name: {customer['name']}")
If struggling, analyze this traceback:
λ python indentation.pyFile "indentation.py", line 7{"name": "Joseph", "lname": "Anderson"},^SyntaxError: invalid syntax
As you can see, the Python interpreter sees the error at the beginning of line 7. The tricky thing here is that this traceback, in fact, translates to a missing comma at the end of line 6. Confused? At first, we were too, but there’s a logical explanation for this.
What is particularly important is that the Python interpreter only points to the place where it first indicated a problem. So if you get a SyntaxError traceback where the code that the traceback is pointing to looks fine, move backwards through the code until you see what’s wrong.
In the example above, there’s clearly no problem with a missing comma in line 7 as it already has one at the end. Put simply, once the interpreter comes to something that doesn’t make sense, it can only direct you to the first thing it found that it couldn’t understand, which is the line before the one indicated by a caret.
Besides, very rarely do we start a code line with a comma. So in cases like this, the caret points not to the actual line with a problem but to the line just before it.
Misspelling keywords
Python keywords are reserved words with a specific meaning. They have a fixed purpose and are always available, so you don’t need to import them into your code. True, false, none, and, for, while, if, not, pass, except – these are just a few examples of very common keywords in Python.
How do those keywords work? For the sake of clarity, let’s take a code example that we’ve already discussed:
10 list = ["item1", "item2", "item3", "item4", "item5"]1112 for item in list:
If you write nothing under the for line, an EOF error will be thrown as the interpreter reaches the end of the file and doesn’t know what to do with it. So if you don't want this statement to do anything, use the “pass” keyword:
12 for item in list:13 pass
Since keywords are a part of the Python programming language, you can only use them in the context that Python allows. If you misspell a keyword in your code, you’ll get a SyntaxError. For example, do you see which keyword is misspelt below?
4 customers = [5 {"name": "Mary", "lname": "Stewart"},6 {"name": "John", "lname": "Doe"},7 {"name": "Joseph", "lname": "Anderson"},8 ]9 10 fro customer in customers:11 print (f"Name: {customer['name']}")12 print (f"Last Name: {customer['name']}")
If you typed the code above, Python would return this traceback:
λ python misspelled_for.pyFile "misspelled_for.py", line 10fro customer in customers:^SyntaxError: invalid syntax
The message says “SyntaxError: invalid syntax” and points to line 10. This is the first place where the interpreter detected that something was wrong. To fix this error, simply write “for” instead of “fro” and run the program again.
And don’t forget that keywords in Python carry clearly defined meanings and can be used only in certain situations. So spelling all of your Python keywords correctly isn’t the only error that developers face. Missing keywords altogether and misusing keywords also occur more often than not.
What is IndentationError?
There’s a sub-class of SyntaxError that deals with indentation issues only. Python indentation is a way of showing that a group of statements belongs to a particular block of code.
The confusion here is that most programming languages like C, C++, and Java use braces { } to denote blocks of code. Python, however, uses whitespaces, i.e. invisible characters like spaces and tabs.
That’s why Python wants whitespaces in your code to behave appropriately, and predictably. As a result, it will raise an IndentationError if the interpreter sees a line in a code block that has the wrong number of spaces.
Have a look at this Python code:
10 list = ["item1", "item2", "item3", "item4", "item5"]1112 for item in list:1314 print (item)
And here’s its traceback:
λ python expected-an-indented-block.pyFile "expected-an-indented-block.py", line 14print (item)^IndentationError: expected an indented block
This is an example of a for loop statement in Python. For loop statements are used to execute a block of statements over and over again until a certain condition is satisfied. The initial loop statement is always followed by a colon (:), while the next line should be indented by 4 spaces.
In the code block above, the line “print (item)” isn’t intended at all. That’s why although the traceback looks like a SyntaxError traceback, it’s an IndentationError. The error message is enormously helpful. It shows that the indentation level of the line “print (item)” doesn’t match any other indentation level.
You can fix this easily by lining up your code lines with the expected indentation level:
12 for item in list:1314 print (item)
This might be extremely difficult to see if a for loop statement has more than one line below. Consider this code block. Any problems with indentation here?
4 customers = [5 {"name": "Mary", "lname": "Stewart"},6 {"name": "John", "lname": "Doe"},7 {"name": "Joseph", "lname": "Anderson"},8 ]910 for customer in customers:11 print (f"Name: {customer['name']}")12 print (f"Last Name: {customer['name']}")
The traceback that we would get after running the above code block is this:
λ python missing_semicolon.pyFile "missing_semicolon.py", line 12print (f"Last Name: {customer['name']}")^IndentationError: unexpected indent
Here, we can clearly see that the last print line in this code is not indented correctly. To fix this issue, make sure that the indentation level of line 12 is the same as that of line 11.
In a nutshell
That’s all, folks! We’ve given you a bunch of common examples of parsing errors in Python and what the solutions to those errors are so that you can understand traceback messages and fix those errors without breaking into a sweat. As we saw, Python tells you what’s wrong with your code, but you can also search for advice online through guides like this one or find a developer community to help you out.
And hey, we know that getting a SyntaxError or an IndentationError might be frustrating. Yet, we’re now confident that next time you get a syntax error, you’ll be better prepared to fix those problems!
So combine your programming skills in Python with our datacenter proxies or residential proxies and scrape the web or parse data easily, successfully, and without limits!
About the author
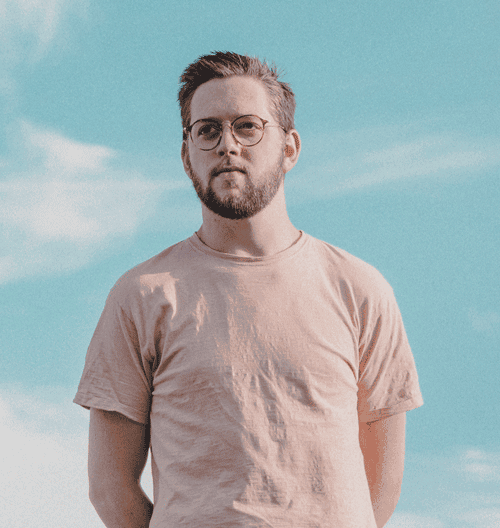
James Keenan
Senior content writer
The automation and anonymity evangelist at Smartproxy. He believes in data freedom and everyone’s right to become a self-starter. James is here to share knowledge and help you succeed with residential proxies.
All information on Smartproxy Blog is provided on an as is basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Smartproxy Blog or any third-party websites that may belinked therein.