Fatal Error: Reached Heap Limit Allocation Failed - JavaScript Heap Out Of Memory
The "Fatal Error: Reached Heap Limit Allocation Failed - JavaScript Heap Out Of Memory" error might seem like a scary mouthful, but understanding it is crucial for optimizing your application's performance and preventing crashes. Think of the JavaScript heap as a bag: pack it too full, and it’ll split at the seams. The error message is your warning that the bag is overloaded and you must make some room or grab a bigger one. Let’s unpack this issue and find out how to make more room for your data.
What is the JavaScript "heap out of memory" error?
A heap is a region of a computer's memory where programs can store and manage data dynamically. This means the program can request memory from the heap and release it when it's no longer needed. It’s a crucial and flexible memory area used to store data that can vary in size and must be managed dynamically throughout a program's execution.
The "Fatal Error: Reached Heap Limit Allocation Failed - JavaScript Heap Out Of Memory" error occurs when a JavaScript application, typically running in a Node.js environment, tries to allocate more memory than is available on the heap. This leads to the application running out of memory, causing the process to crash.
What causes the JavaScript “heap out of memory” fatal error?
By default, the maximum default heap size in Node.js (up to 11.x) is 700 MB on 32-bit and 1400 MB on 64-bit platforms. Therefore, if your application exceeds this limit, you’ll run into the "heap out of memory" error. But why does this happen? There are several factors:
- Large data structures. Collections of data that occupy a significant amount of memory during its execution can cause memory issues. These can be massive arrays with many elements, complex objects with many properties, or large files and datasets like images, videos, JSON, or CSV files.
- Memory leaks. A memory leak occurs when a program allocates memory during execution but fails to release it when it's no longer needed. It must be noted that it can be done unintentionally, especially in languages like JavaScript that release unused memory automatically. However, if objects are kept in memory by lingering references (such as global variables or event listeners), they won’t be garbage collected, leading to a memory leak.
- Inefficient code. Algorithms or functions that use more resources than necessary to accomplish a task. The importance of efficient code might not always be clear to beginner or even advanced programmers, but it can quickly lead to optimization issues or cause errors. Here’s an example:
var data = {0: 'item 1',1: 'item 2',2: 'item 3',// ...};
Instead of using an array, the values are stored in an object. A method like this is very complicated for no reason and uses more computer resources than needed.
- Recursive functions. A function that calls itself to solve a problem. Recursion is a common technique used to break down complex problems into simpler, more manageable parts. For example, calculating the factorial of a number or traversing a tree data structure. Each time a recursive function calls itself, the current state of the function is pushed onto the call stack. Do this enough times, and you’re going to overload it, causing a stack overflow (that’s where the name of our favorite website comes from!). Here’s an example code that may cause this issue:
function factorial(n) {if (n === 0) {return 1;} else {return n * factorial(n - 1);}}const result = factorial(100000);console.log(result)
- High concurrent requests. In a server environment, these refer to multiple requests being handled at the same time. When a server receives a high number of requests at the same time, it can strain the server's resources. It’s like trying to answer multiple messages on Slack at the same time while getting pings from the group chat, while a colleague walks up behind you to ask a question, while you’re getting a phone call. Wowee.
In simple terms, the "heap out of memory" error occurs when you ask the computer to do more than it can. While computers are efficient and powerful in the modern age, they still have set limitations. Errors like these are simply there to prevent issues that could cause stability or damage to your machine.
How to fix the JavaScript "heap out of memory" error?
If you got this error and feel like you reached a dead end, don’t worry, as there are several solutions to this problem. All you need to do is enter a simple command that increases the memory limit or make a few adjustments to your code or the dataset.
Increase the memory limit
The most straightforward way to overcome this error is to simply increase the set memory limit. As mentioned before, Node.js has a memory limit of around 1.5 GB. You can increase this limit by passing the --max-old-space-size flag when running your Node.js application. This flag allows you to specify the maximum memory size (in megabytes) that Node.js can allocate:
node --max-old-space-size=5000 your-script.js
Alternatively, if you don’t want to set the maximum size every time you run a script, you can export it as an environment variable:
export NODE_OPTIONS=--max_old_space_size=5000
The above commands allow you to run your script with a new memory limit of 5000 MB (5 GB). You can change the number to whichever value you desire, but take care not to go crazy with power and put stress on your computer.
Optimize your code
One of the best ways to avoid an issue is to not cause it in the first place. Seems logical enough, right? A clean and optimized code should be the standard for any self-respecting developer because it increases the application performance and avoids "heap out of memory" errors.
Here are a few suggestions to help you optimize your code:
- Minimize global variables. Global variables stay in memory the entire time the application is being used. If you have large objects or arrays stored globally, they might unnecessarily occupy memory even when they’re not in use. Therefore, instead of writing the code like this:
let largeData; // Declares the variable at a global scopefunction loadData() {largeData = fetchData();}
You can do it in a more optimal way:
function loadData() {const largeData = fetchData(); // Declares the variable at a local scope, which is released after function executionprocessLargeData(largeData);}
- Identify memory hotspots. Identifying parts of your code that consume the most memory is crucial for optimization. You can start your Node.js application with the --inspect flag and use tools like Chrome DevTools to monitor memory usage and identify areas where memory consumption is abnormally high. You can then go back to those specific areas of your code and figure out how to make them more efficient.
node --inspect your-script.js
- Offload processing to external services. Prevent the main application from running out of memory by delegating heavy lifting to other systems. For example, if your application needs to process large images or videos, use external services or APIs designed for such tasks. Services like AWS Lambda, Google Cloud Functions, or dedicated image processing services can handle these tasks without consuming your application's memory.
You probably can optimize your code in countless ways – we’ve named just a few of them. Always stick to best coding practices, write clean and efficient code, and perform regular audits. This way, you’ll avoid any issues that may arise.
Split large datasets
When working with large datasets, such as reading from a database or processing large files, loading all the data into memory at once can easily exhaust available memory. Instead, use pagination or batching to process smaller chunks of data sequentially.
Here’s an example of how you can use streams to process data in chunks Instead of loading an entire file or dataset into memory:
const fs = require('fs');const readline = require('readline');// Create a readable stream from the large file “largeFile.txt”. This will read the file in small chunks, rather than loading the entire file into memory.const fileStream = fs.createReadStream('largeFile.txt');// Create an interface for reading the file line by lineconst rl = readline.createInterface({input: fileStream,crlfDelay: Infinity});rl.on('line', (line) => {console.log(line);});
The above code ensures that the file is read and processed line-by-line instead of being loaded in its entirety. You can use this method to read certain lines from a file, perform various operations on them, and only then move to the next line without hogging up the heap memory.
You may also want to optimize the datasets that you have before they’re handled by your application. This includes cleaning the data, checking for duplicates, aggregating similar entries, or compressing it to take up less storage space. A neatly organized dataset can save you hours of trouble later down the line.
If you’re curious about where to obtain well-structured and easy-to-manage data for your project, check out Smartproxy’s Scraping APIs. These APIs allow easy and unrestricted access to various websites, eCommerce pages, and search results from Google, Bing, and many more. The data can then be downloaded in widely supported CSV, JSON, table, or HTML formats, ensuring that management is simple and efficient.
How to prevent the "fatal error: JavaScript heap out of memory" error in the future?
The best way to prevent the "heap out of memory" error from happening in your future projects is by proactively avoiding it. Here’s a summary of the above-mentioned tips to keep in mind:
- Increase the memory limit. By increasing the memory limit available to your Node.js process, you give your application more room to handle large datasets, complex operations, or high volumes of concurrent requests without crashing due to memory constraints.
- Optimize your code. Writing efficient code can significantly reduce memory usage, making your application less prone to hitting memory limits.
- Use clean datasets. Datasets that are cluttered with duplicates, irrelevant data, or inconsistencies can waste valuable memory and processing time. Clean datasets are leaner, more accurate, and easier to manage, reducing the likelihood of memory issues.
Final words
Encountering the "heap out of memory" error in JavaScript can be a daunting experience, but it also presents an opportunity to refine your approach to coding and memory management. You can significantly reduce the risk of running into this issue by increasing memory limits where necessary, optimizing your code to be more efficient, and ensuring that your datasets are clean and well-structured.
References
You Don't Know JS (YDKJS) by Kyle Simpson
Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
About the author
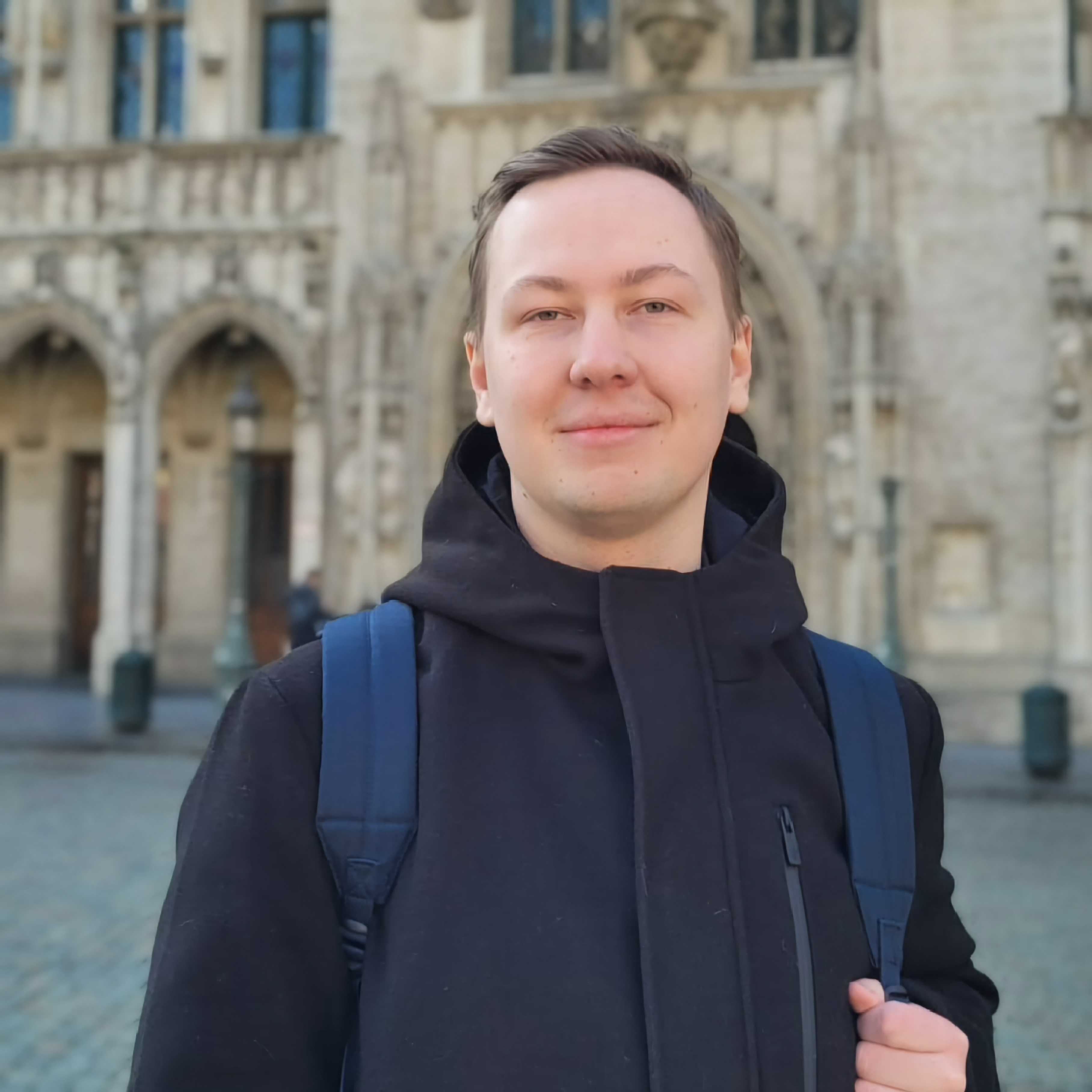
Zilvinas Tamulis
Technical Copywriter
Zilvinas is an experienced technical copywriter specializing in web development and network technologies. With extensive proxy and web scraping knowledge, he’s eager to share valuable insights and practical tips for confidently navigating the digital world.
All information on Smartproxy Blog is provided on an as is basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Smartproxy Blog or any third-party websites that may belinked therein.