The Ultimate Guide to Web Scraping Job Postings with Python in 2024
Did you know that there are thousands of job postings scattered across different websites and platforms, making it nearly impossible to keep track of all the opportunities out there? Thankfully, with the power of web scraping and the versatility of Python, you can automate this tedious job search process and land your dream job faster than ever.
How to scrape job postings with Python in 5 steps
Web scraping job postings with Python involves automating data extraction from various websites to gather job listings efficiently. Here’s a step-by-step process to help you get started:
- Step 1 – identify your data needs. Determine what information you want to extract, such as job titles, companies, locations, and job descriptions. This will guide your scraping process.
- Step 2 – set up your web scraping tool. Install Python and essential libraries like BeautifulSoup, Scrapy, Requests, etc. Configure your coding environment using an IDE like PyCharm or Visual Studio Code.
- Step 3 – Write your first web scraping script. Here's an example of what a simple script can look like:
import requestsfrom bs4 import BeautifulSoup# Send a GET request to the websiteurl = 'https://example.com/jobs' # Replace with the actual URLresponse = requests.get(url)# Send a GET request to the websiteurl = 'https://example.com/jobs' # Replace with the actual URLresponse = requests.get(url)# Parse the HTML content using BeautifulSoupsoup = BeautifulSoup(response.text, 'html.parser')# Select job titles and company names using the appropriate CSS selectorsjob_titles = soup.select('.job-title') # Adjust the selector based on the site's structurecompany_names = soup.select('.company-name') # Adjust the selector based on the site's structure# Iterate through both lists of job titles and company namesfor title, company in zip(job_titles, company_names):print(f"Job Title: {title.get_text(strip=True)}")print(f"Company Name: {company.get_text(strip=True)}\n")
- Step 4 – handle pagination. Loop through multiple pages to gather all job listings.
- Step 5 – handle dynamic content. You can use tools like Selenium to interact with websites that load content with JavaScript.
By following these steps, you can efficiently scrape job postings from multiple websites, making your job search more streamlined and effective.
Getting started with Python for web scraping
Now that we understand the importance of web scraping let's dive into why Python is the perfect programming language for this task. Python has a rich ecosystem of libraries and frameworks specifically designed for web scraping, making it incredibly intuitive and convenient to work with.
Not only is Python widely adopted by developers, but it also offers powerful tools such as BeautifulSoup and Scrapy, which simplify the process of extracting data from websites. These libraries provide a wide range of features, enabling you to:
- navigate web pages
- select specific elements
- extract the desired information with just a few lines of code
Python's popularity in the web scraping community isn't without reason. Its versatility allows you to tackle various scraping tasks, from simple data extraction to complex web crawling.
With Python, you can easily handle different data types, including HTML, XML, JSON, and more. This flexibility gives you the freedom to scrape information from various sources and formats, making Python an invaluable tool for any web scraping project.
Writing your first web scraping script with Python
Before we dive into coding, it's important to understand the structure of a web page. By analyzing the HTML structure of the page, we can identify the elements that contain the job postings we're interested in.
Understanding the structure of a web page
When inspecting a web page, right-click on any element and select Inspect to open the browser's developer tools. This will display the HTML structure of the page, allowing you to navigate through the elements and identify the ones that contain the job postings.
For example, let's say you're interested in scraping job postings from a popular job search website.
By inspecting the HTML structure, you might find that job titles are contained within a website. The <h2> element might have a class named "job-title", and the company names are within an <span> element with the class "company-name". With this information, you can confidently proceed to write your web scraping script, targeting these specific elements to extract the desired data.
Writing a basic Python script for web scraping
Now that we understand the structure of a web page, let's write a basic Python script to scrape job postings. Using the BeautifulSoup library, we can easily extract the desired information from the HTML response.
First, we'll need to import the necessary libraries:
import requestsfrom bs4 import BeautifulSoup
Next, we'll send an HTTP request to the website containing the job postings and retrieve the HTML response:
url = 'https://www.example.com/job-postings'response = requests.get(url)
Once we have the HTML response, we can create a BeautifulSoup object to parse the HTML and extract the desired information. Let's say we're interested in the job titles and company names:
soup = BeautifulSoup(response.text, 'html.parser')job_titles = soup.select('.job-title')company_names = soup.select('.company-name')# Iterating through both lists of job titles and company namesfor title, company in zip(job_titles, company_names):print(f'Job Title: {title.text.strip()}')print(f'Company: {company.text.strip()}')print() # Print a blank line for separation between job listings
With just a few lines of code, we're now able to scrape job titles and company names from a web page. Of course, this is just the tip of the iceberg when it comes to web scraping.
Advanced methods
Let's dive into some advanced techniques to take our web scraping skills to the next level.
One advanced technique is to handle pagination. Many websites display job postings across multiple pages. You'll need to navigate the pages and extract the information from each page to scrape all the job postings. This can be achieved by identifying the pagination elements in the HTML structure and dynamically generating the URLs for each page.
Another technique is to handle dynamic content. Some websites load content dynamically using JavaScript. This means the initial HTML response may not contain all the job postings. To scrape these dynamic job postings, you'll need to use tools like Selenium to automate the interaction with the website and retrieve the updated HTML response.
Common challenges in web scraping with Python
As we become more proficient in web scraping, we may encounter more complex scenarios that require advanced techniques. Here are a couple of challenges you might encounter and how to overcome them:
Handling pagination and dynamic content
Many websites paginate their job listings, meaning that you'll need to navigate through multiple pages to gather all the information. To handle pagination, you can create a loop that iterates through the pages, extracting the desired data from each page.
But what if the website you're scraping has dynamic content loaded using JavaScript? The content you're looking for might not be in the initial HTML response. This can be a real challenge, but fear not! There's a solution.
One way to handle dynamic content is by using a powerful Selenium tool. Selenium allows you to interact with the website as if you were a real user, enabling you to access the dynamically loaded content. With Selenium, you can automate actions like clicking buttons, filling out forms, and scrolling through the page to ensure you capture all the data you need.
Dealing with CAPTCHAs and login forms
Some websites implement CAPTCHAs or require user authentication to access their job postings. CAPTCHAs, those pesky little tests designed to differentiate humans from bots, can be a major roadblock in your web scraping journey.
One option to overcome this is to use services like proxies, which can help avoid getting CAPTCHAs in the first place. Another way is to use services like AntiCaptcha, which can automatically solve CAPTCHAs for you. These services employ advanced algorithms to analyze and solve CAPTCHAs, saving you valuable time and effort. Alternatively, you can also solve CAPTCHAs manually using Selenium. You can streamline your web scraping workflow by automating the process of solving CAPTCHAs.
Now, what if the website you're scraping requires user authentication? In such cases, you must include the necessary credentials in your script to log in before scraping the data. This can be achieved by sending POST requests with the login information or using Selenium to automate the login process. You can access the restricted content and extract the desired data by providing the required credentials.
Remember, the key to successful web scraping is adapting to the unique challenges presented by each website. By combining your programming skills with a deep understanding of HTML structure and web page dynamics, you'll be able to tackle any scraping project that comes your way.
Your next steps: master web scraping with Python
So why not dive into the world of web scraping and see how it can supercharge your job hunt? Whether you're a seasoned programmer or just starting your coding journey, web scraping opens up a world of opportunities by automating the job search process.
With the ultimate guide to web scraping job postings with Python in your hands, you have the tools to take your job search to the next level. Happy scraping!
About the author
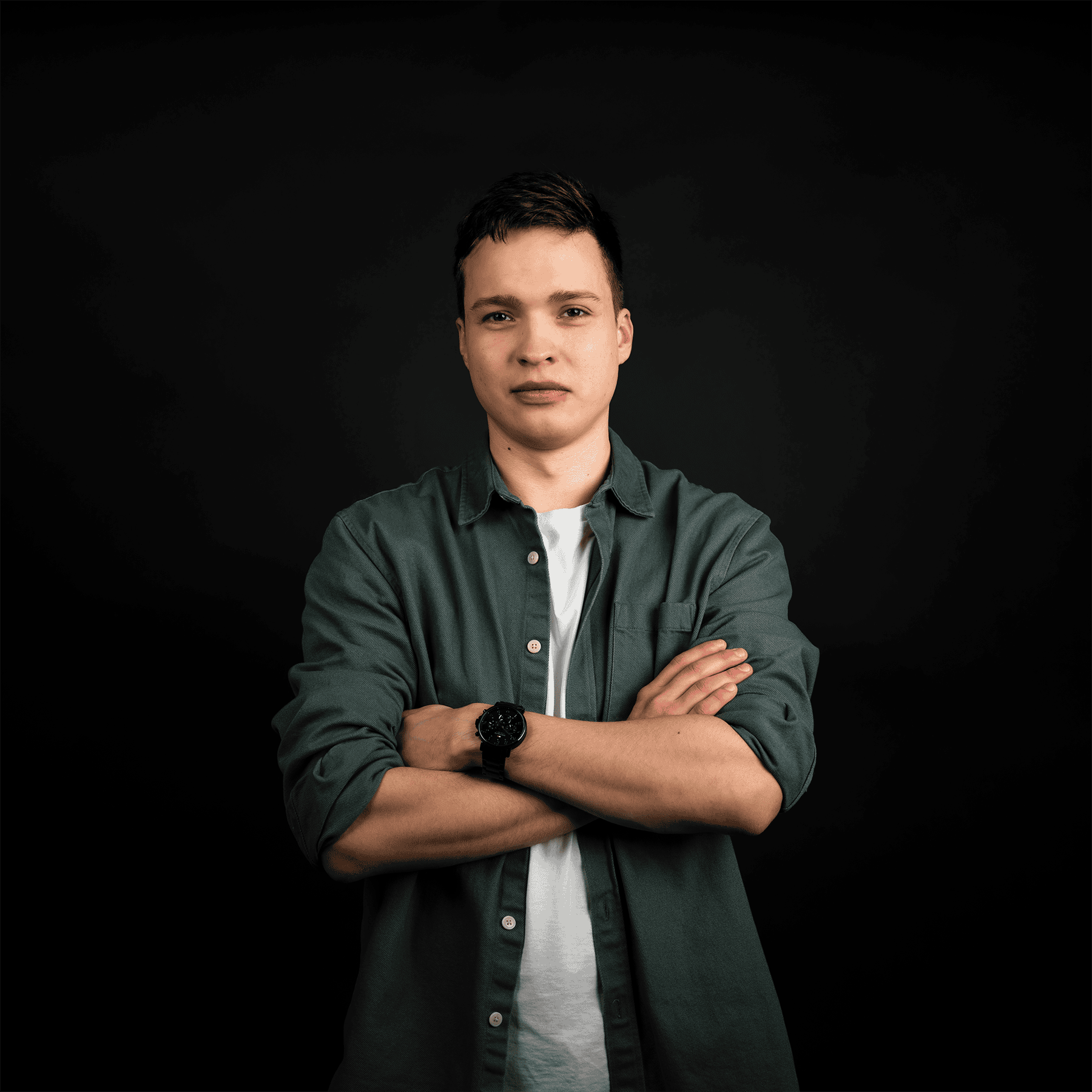
Vilius Sakutis
Head of Partnerships
With an eagerness to create beneficial partnerships that drive business growth, Vilius brings valuable expertise and collaborative spirit to the table. His skill set is a valuable asset for those seeking to uncover new possibilities and learn more about the proxy market.
All information on Smartproxy Blog is provided on an as is basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Smartproxy Blog or any third-party websites that may belinked therein.